はじめに
Laravelは、メール送信のサポートに加えて、メール、SMS(Vonage、以前はNexmoとして知られていた)、およびSlackを含むさまざまな配信チャネルを通じて通知を送信するサポートを提供します。さらに、数十の異なるチャネルを介して通知を送信するために作成されたさまざまなコミュニティ構築の通知チャネルもあります!通知はデータベースに保存され、ウェブインターフェースに表示されることもあります。
通常、通知はアプリケーション内で発生した何かをユーザーに通知する短い情報メッセージであるべきです。たとえば、請求アプリケーションを作成している場合、ユーザーに「請求書が支払われました」という通知をメールおよびSMSチャネルを介して送信することができます。
通知の生成
Laravelでは、各通知は通常app/Notifications
ディレクトリに保存される単一のクラスで表されます。このディレクトリがアプリケーションに表示されない場合でも心配しないでください。make:notification
Artisanコマンドを実行すると、自動的に作成されます:
php artisan make:notification InvoicePaid
このコマンドは、app/Notifications
ディレクトリに新しい通知クラスを配置します。各通知クラスには、via
メソッドと、toMail
やtoDatabase
などのメッセージ構築メソッドが含まれており、特定のチャネルに合わせたメッセージに通知を変換します。
通知の送信
Notifiableトレイトの使用
通知は2つの方法で送信できます:notify
メソッドを使用するNotifiable
トレイトまたはNotification
ファサードを使用します。Notifiable
トレイトは、アプリケーションのApp\Models\User
モデルにデフォルトで含まれています:
<?php
namespace App\Models;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use Notifiable;
}
このトレイトによって提供されるnotify
メソッドは、通知インスタンスを受け取ることを期待しています:
use App\Notifications\InvoicePaid;
$user->notify(new InvoicePaid($invoice));
覚えておいてください、Notifiable
トレイトはあなたのモデルのいずれかに使用できます。User
モデルにのみ含める必要はありません。
通知ファサードの使用
また、Notification
ファサードを介して通知を送信することもできます。このアプローチは、ユーザーのコレクションなど、複数の通知可能なエンティティに通知を送信する必要がある場合に便利です。ファサードを使用して通知を送信するには、すべての通知可能なエンティティと通知インスタンスをsend
メソッドに渡します:
use Illuminate\Support\Facades\Notification;
Notification::send($users, new InvoicePaid($invoice));
``````php
Notification::sendNow($developers, new DeploymentCompleted($deployment));
`
配信チャネルの指定
すべての通知クラスには、通知が配信されるチャネルを決定するvia
メソッドがあります。通知はmail
、database
、broadcast
、vonage
、およびslack
チャネルで送信できます。
TelegramやPusherなどの他の配信チャネルを使用したい場合は、コミュニティ主導のLaravel Notification Channelsウェブサイトを確認してください。
``````php
/**
* Get the notification's delivery channels.
*
* @return array<int, string>
*/
public function via(object $notifiable): array
{
return $notifiable->prefers_sms ? ['vonage'] : ['mail', 'database'];
}
`
通知のキューイング
通知をキューに入れる前に、キューを構成し、ワーカーを開始する必要があります。
通知の送信には時間がかかる場合があります。特に、チャネルが通知を配信するために外部API呼び出しを行う必要がある場合です。アプリケーションの応答時間を短縮するために、ShouldQueue
インターフェースとQueueable
トレイトをクラスに追加して、通知をキューに入れさせることができます。インターフェースとトレイトは、make:notification
コマンドを使用して生成されたすべての通知に対してすでにインポートされているため、すぐに通知クラスに追加できます:
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Notification;
class InvoicePaid extends Notification implements ShouldQueue
{
use Queueable;
// ...
}
``````php
$user->notify(new InvoicePaid($invoice));
`
通知をキューに入れると、各受信者とチャネルの組み合わせに対してキューされたジョブが作成されます。たとえば、通知に3人の受信者と2つのチャネルがある場合、6つのジョブがキューに送信されます。
通知の遅延
通知の配信を遅延させたい場合は、通知のインスタンス化にdelay
メソッドをチェーンすることができます:
$delay = now()->addMinutes(10);
$user->notify((new InvoicePaid($invoice))->delay($delay));
特定のチャネルの遅延量を指定するために、delay
メソッドに配列を渡すことができます:
$user->notify((new InvoicePaid($invoice))->delay([
'mail' => now()->addMinutes(5),
'sms' => now()->addMinutes(10),
]));
または、通知クラス自体にwithDelay
メソッドを定義することもできます。withDelay
メソッドは、チャネル名と遅延値の配列を返す必要があります:
/**
* Determine the notification's delivery delay.
*
* @return array<string, \Illuminate\Support\Carbon>
*/
public function withDelay(object $notifiable): array
{
return [
'mail' => now()->addMinutes(5),
'sms' => now()->addMinutes(10),
];
}
通知キュー接続のカスタマイズ
デフォルトでは、キューされた通知はアプリケーションのデフォルトキュー接続を使用してキューに入れられます。特定の通知に使用する別の接続を指定したい場合は、通知のコンストラクタからonConnection
メソッドを呼び出すことができます:
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Notification;
class InvoicePaid extends Notification implements ShouldQueue
{
use Queueable;
/**
* Create a new notification instance.
*/
public function __construct()
{
$this->onConnection('redis');
}
}
または、通知がサポートする各通知チャネルに使用する特定のキュー接続を指定したい場合は、通知にviaConnections
メソッドを定義できます。このメソッドは、チャネル名/キュー接続名のペアの配列を返す必要があります:
/**
* Determine which connections should be used for each notification channel.
*
* @return array<string, string>
*/
public function viaConnections(): array
{
return [
'mail' => 'redis',
'database' => 'sync',
];
}
通知チャネルキューのカスタマイズ
通知がサポートする各通知チャネルに使用する特定のキューを指定したい場合は、通知にviaQueues
メソッドを定義できます。このメソッドは、チャネル名/キュー名のペアの配列を返す必要があります:
/**
* Determine which queues should be used for each notification channel.
*
* @return array<string, string>
*/
public function viaQueues(): array
{
return [
'mail' => 'mail-queue',
'slack' => 'slack-queue',
];
}
キューされた通知ミドルウェア
キューされた通知は、キューされたジョブと同様にミドルウェアを定義できます。始めるには、通知クラスにmiddleware
メソッドを定義します。middleware
メソッドは、$notifiable
および$channel
変数を受け取り、通知の宛先に基づいて返されるミドルウェアをカスタマイズできます:
use Illuminate\Queue\Middleware\RateLimited;
/**
* Get the middleware the notification job should pass through.
*
* @return array<int, object>
*/
public function middleware(object $notifiable, string $channel)
{
return match ($channel) {
'email' => [new RateLimited('postmark')],
'slack' => [new RateLimited('slack')],
default => [],
};
}
キューされた通知とデータベーストランザクション
キューされた通知がデータベーストランザクション内で送信されると、データベーストランザクションがコミットされる前にキューによって処理される場合があります。この場合、データベーストランザクション中にモデルやデータベースレコードに加えた更新がデータベースにまだ反映されていない可能性があります。さらに、トランザクション内で作成されたモデルやデータベースレコードは、データベースに存在しない可能性があります。通知がこれらのモデルに依存している場合、キューされた通知を送信するジョブが処理されるときに予期しないエラーが発生する可能性があります。
キュー接続のafter_commit
構成オプションがfalse
に設定されている場合でも、特定のキューされた通知がすべてのオープンデータベーストランザクションがコミットされた後に送信されるべきであることを示すことができます。通知を送信するときにafterCommit
メソッドを呼び出します:
use App\Notifications\InvoicePaid;
$user->notify((new InvoicePaid($invoice))->afterCommit());
または、通知のコンストラクタからafterCommit
メソッドを呼び出すこともできます:
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Notification;
class InvoicePaid extends Notification implements ShouldQueue
{
use Queueable;
/**
* Create a new notification instance.
*/
public function __construct()
{
$this->afterCommit();
}
}
これらの問題を回避する方法について詳しくは、キューされたジョブとデータベーストランザクションに関するドキュメントを確認してください。
キューされた通知を送信するかどうかの判断
キューされた通知がバックグラウンド処理のためにキューに送信されると、通常はキュー作業者によって受け入れられ、意図された受信者に送信されます。
ただし、キュー作業者によって処理された後にキューされた通知を送信するかどうかの最終的な判断を行いたい場合は、通知クラスにshouldSend
メソッドを定義できます。このメソッドがfalse
を返す場合、通知は送信されません:
/**
* Determine if the notification should be sent.
*/
public function shouldSend(object $notifiable, string $channel): bool
{
return $this->invoice->isPaid();
}
オンデマンド通知
時には、アプリケーションの「ユーザー」として保存されていない誰かに通知を送信する必要があるかもしれません。Notification
ファサードのroute
メソッドを使用して、通知を送信する前にアドホックな通知ルーティング情報を指定できます:
use Illuminate\Broadcasting\Channel;
use Illuminate\Support\Facades\Notification;
Notification::route('mail', '')
->route('vonage', '5555555555')
->route('slack', '#slack-channel')
->route('broadcast', [new Channel('channel-name')])
->notify(new InvoicePaid($invoice));
オンデマンド通知をmail
ルートに送信する際に受信者の名前を提供したい場合は、メールアドレスをキーとし、配列の最初の要素の値として名前を含む配列を提供できます:
Notification::route('mail', [
'' => 'Barrett Blair',
])->notify(new InvoicePaid($invoice));
``````php
Notification::routes([
'mail' => ['' => 'Barrett Blair'],
'vonage' => '5555555555',
])->notify(new InvoicePaid($invoice));
`
メール通知
メールメッセージのフォーマット
通知がメールとして送信されることをサポートしている場合、通知クラスにtoMail
メソッドを定義する必要があります。このメソッドは、$notifiable
エンティティを受け取り、Illuminate\Notifications\Messages\MailMessage
インスタンスを返す必要があります。
``````php
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
$url = url('/invoice/'.$this->invoice->id);
return (new MailMessage)
->greeting('Hello!')
->line('One of your invoices has been paid!')
->lineIf($this->amount > 0, "Amount paid: {$this->amount}")
->action('View Invoice', $url)
->line('Thank you for using our application!');
}
`
この例では、挨拶、テキストの行、アクションを呼びかける部分、そしてもう一つのテキストの行を登録します。`````MailMessage`````オブジェクトによって提供されるこれらのメソッドは、小さなトランザクションメールをフォーマットするのを簡単かつ迅速にします。メールチャネルは、メッセージコンポーネントを美しい、レスポンシブなHTMLメールテンプレートに変換し、プレーンテキストの対応物を自動的に生成します。`````mail`````チャネルによって生成されたメールの例は次のとおりです:
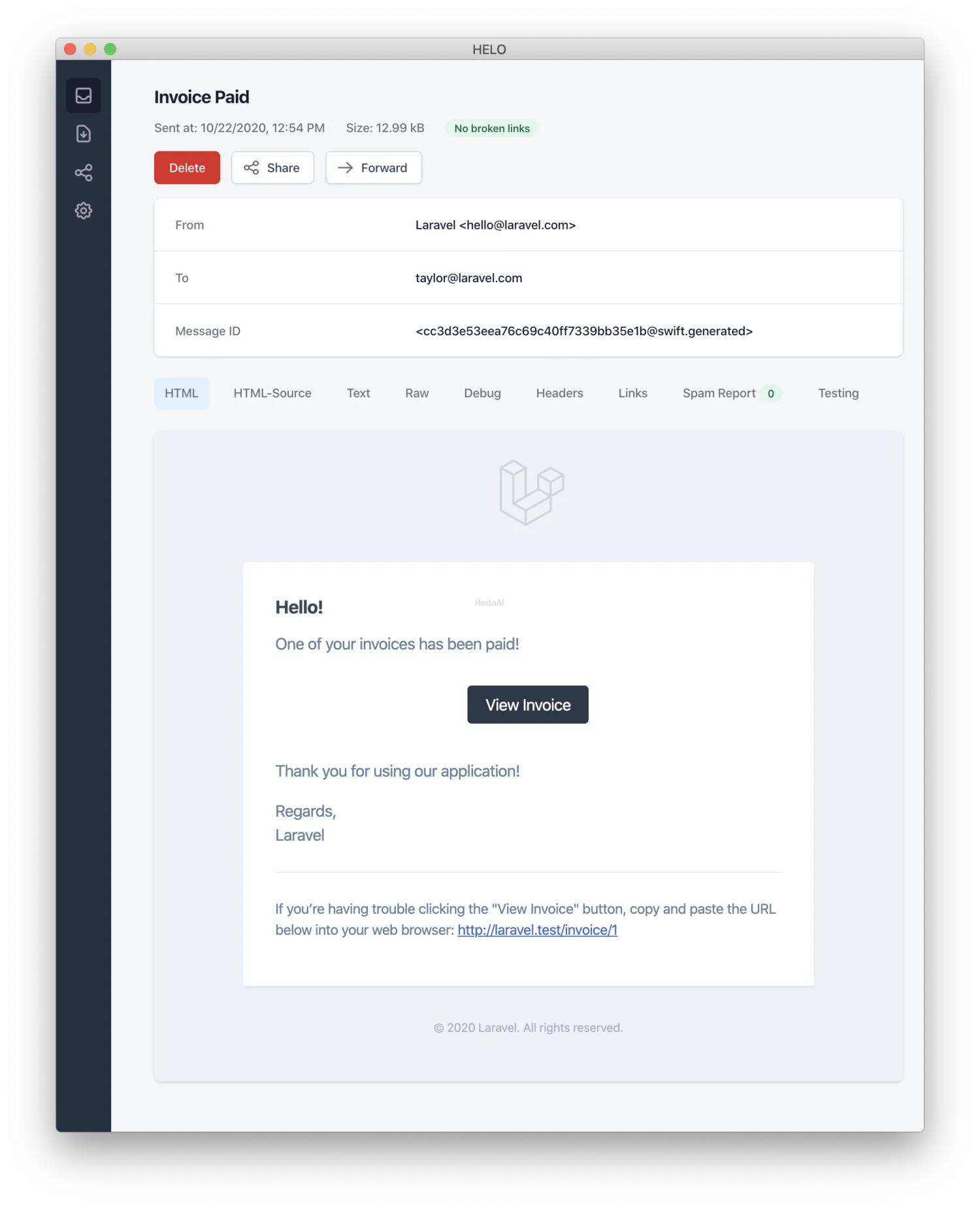
メール通知を送信する際は、`````name`````構成オプションを`````config/app.php`````構成ファイルに設定してください。この値は、メール通知メッセージのヘッダーとフッターに使用されます。
<a name="error-messages"></a>
#### エラーメッセージ
一部の通知は、失敗した請求書の支払いなど、ユーザーにエラーを通知します。メッセージを構築する際に`````error`````メソッドを呼び出すことで、メールメッセージがエラーに関するものであることを示すことができます。メールメッセージの`````error`````メソッドを使用すると、アクションを呼びかけるボタンが黒ではなく赤になります:
``````php
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->error()
->subject('Invoice Payment Failed')
->line('...');
}
`
その他のメール通知フォーマットオプション
通知クラスで「行」を定義する代わりに、view
メソッドを使用して通知メールをレンダリングするために使用されるカスタムテンプレートを指定できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)->view(
'mail.invoice.paid', ['invoice' => $this->invoice]
);
}
メールメッセージのプレーンテキストビューを指定するには、view
メソッドに渡される配列の2番目の要素としてビュー名を渡します:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)->view(
['mail.invoice.paid', 'mail.invoice.paid-text'],
['invoice' => $this->invoice]
);
}
または、メッセージにプレーンテキストビューしかない場合は、text
メソッドを利用できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)->text(
'mail.invoice.paid-text', ['invoice' => $this->invoice]
);
}
送信者のカスタマイズ
デフォルトでは、メールの送信者/送信元アドレスはconfig/mail.php
構成ファイルで定義されています。ただし、特定の通知の送信元アドレスをfrom
メソッドを使用して指定できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->from('', 'Barrett Blair')
->line('...');
}
受信者のカスタマイズ
``````php
<?php
namespace App\Models;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Illuminate\Notifications\Notification;
class User extends Authenticatable
{
use Notifiable;
/**
* Route notifications for the mail channel.
*
* @return array<string, string>|string
*/
public function routeNotificationForMail(Notification $notification): array|string
{
// Return email address only...
return $this->email_address;
// Return email address and name...
return [$this->email_address => $this->name];
}
}
`
件名のカスタマイズ
デフォルトでは、メールの件名は「タイトルケース」にフォーマットされた通知のクラス名です。したがって、通知クラスの名前がInvoicePaid
の場合、メールの件名はInvoice Paid
になります。メッセージの異なる件名を指定したい場合は、メッセージを構築する際にsubject
メソッドを呼び出すことができます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->subject('Notification Subject')
->line('...');
}
メール送信者のカスタマイズ
デフォルトでは、メール通知はconfig/mail.php
構成ファイルで定義されたデフォルトのメール送信者を使用して送信されます。ただし、メッセージを構築する際にmailer
メソッドを呼び出すことで、実行時に異なるメール送信者を指定できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->mailer('postmark')
->line('...');
}
テンプレートのカスタマイズ
メール通知で使用されるHTMLおよびプレーンテキストテンプレートを変更するには、通知パッケージのリソースを公開します。このコマンドを実行した後、メール通知テンプレートはresources/views/vendor/notifications
ディレクトリに配置されます:
php artisan vendor:publish --tag=laravel-notifications
添付ファイル
メール通知に添付ファイルを追加するには、メッセージを構築する際にattach
メソッドを使用します。attach
メソッドは、ファイルへの絶対パスを最初の引数として受け取ります:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->greeting('Hello!')
->attach('/path/to/file');
}
通知メールメッセージによって提供されるattach
メソッドも添付可能なオブジェクトを受け入れます。詳細については、包括的な添付可能オブジェクトのドキュメントを参照してください。
メッセージにファイルを添付する際、表示名および/またはMIMEタイプを指定することもできます。これは、array
をattach
メソッドの2番目の引数として渡すことで行います:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->greeting('Hello!')
->attach('/path/to/file', [
'as' => 'name.pdf',
'mime' => 'application/pdf',
]);
}
メールオブジェクトにファイルを添付するのとは異なり、attachFromStorage
を使用してストレージディスクから直接ファイルを添付することはできません。代わりに、ストレージディスク上のファイルへの絶対パスを使用してattach
メソッドを使用する必要があります。あるいは、toMail
メソッドからmailableを返すこともできます:
use App\Mail\InvoicePaid as InvoicePaidMailable;
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): Mailable
{
return (new InvoicePaidMailable($this->invoice))
->to($notifiable->email)
->attachFromStorage('/path/to/file');
}
必要に応じて、attachMany
メソッドを使用してメッセージに複数のファイルを添付できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->greeting('Hello!')
->attachMany([
'/path/to/forge.svg',
'/path/to/vapor.svg' => [
'as' => 'Logo.svg',
'mime' => 'image/svg+xml',
],
]);
}
生データの添付
``````php
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->greeting('Hello!')
->attachData($this->pdf, 'name.pdf', [
'mime' => 'application/pdf',
]);
}
`
タグとメタデータの追加
MailgunやPostmarkなどの一部のサードパーティのメールプロバイダーは、メッセージの「タグ」と「メタデータ」をサポートしており、これを使用してアプリケーションから送信されたメールをグループ化および追跡できます。tag
およびmetadata
メソッドを介して、メールメッセージにタグとメタデータを追加できます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->greeting('Comment Upvoted!')
->tag('upvote')
->metadata('comment_id', $this->comment->id);
}
アプリケーションがMailgunドライバーを使用している場合は、タグおよびメタデータに関する詳細情報についてMailgunのドキュメントを参照してください。同様に、Postmarkのドキュメントもタグおよびメタデータのサポートに関する詳細情報を参照できます。
アプリケーションがAmazon SESを使用してメールを送信している場合は、metadata
メソッドを使用してメッセージにSES「タグ」を添付する必要があります。
Symfonyメッセージのカスタマイズ
``````php
use Symfony\Component\Mime\Email;
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->withSymfonyMessage(function (Email $message) {
$message->getHeaders()->addTextHeader(
'Custom-Header', 'Header Value'
);
});
}
`
Mailablesの使用
必要に応じて、通知のtoMail
メソッドから完全なmailableオブジェクトを返すことができます。MailMessage
の代わりにMailable
を返す場合、mailableオブジェクトのto
メソッドを使用してメッセージの受信者を指定する必要があります:
use App\Mail\InvoicePaid as InvoicePaidMailable;
use Illuminate\Mail\Mailable;
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): Mailable
{
return (new InvoicePaidMailable($this->invoice))
->to($notifiable->email);
}
Mailablesとオンデマンド通知
オンデマンド通知を送信している場合、$notifiable
メソッドに渡されるインスタンスはtoMail
のインスタンスであり、routeNotificationFor
メソッドを使用してオンデマンド通知を送信するメールアドレスを取得できます:
use App\Mail\InvoicePaid as InvoicePaidMailable;
use Illuminate\Notifications\AnonymousNotifiable;
use Illuminate\Mail\Mailable;
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): Mailable
{
$address = $notifiable instanceof AnonymousNotifiable
? $notifiable->routeNotificationFor('mail')
: $notifiable->email;
return (new InvoicePaidMailable($this->invoice))
->to($address);
}
メール通知のプレビュー
メール通知テンプレートを設計する際、通常のBladeテンプレートのようにブラウザでレンダリングされたメールメッセージをすぐにプレビューできるのは便利です。このため、Laravelは、メール通知によって生成された任意のメールメッセージをルートクロージャまたはコントローラから直接返すことを許可します。MailMessage
が返されると、それはレンダリングされ、ブラウザに表示され、実際のメールアドレスに送信することなくデザインをすぐにプレビューできます:
use App\Models\Invoice;
use App\Notifications\InvoicePaid;
Route::get('/notification', function () {
$invoice = Invoice::find(1);
return (new InvoicePaid($invoice))
->toMail($invoice->user);
});
Markdownメール通知
Markdownメール通知を使用すると、メール通知の事前構築されたテンプレートを活用しながら、より長くカスタマイズされたメッセージを書く自由が得られます。メッセージはMarkdownで書かれているため、Laravelはメッセージの美しいレスポンシブHTMLテンプレートをレンダリングし、プレーンテキストの対応物も自動的に生成できます。
メッセージの生成
対応するMarkdownテンプレートを持つ通知を生成するには、--markdown
オプションを使用してmake:notification
Artisanコマンドを実行します:
php artisan make:notification InvoicePaid --markdown=mail.invoice.paid
他のすべてのメール通知と同様に、Markdownテンプレートを使用する通知は、通知クラスにtoMail
メソッドを定義する必要があります。ただし、通知を構築するためにline
およびaction
メソッドを使用するのではなく、markdown
メソッドを使用して使用するMarkdownテンプレートの名前を指定します。テンプレートに利用可能にしたいデータの配列をメソッドの2番目の引数として渡すことができます:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
$url = url('/invoice/'.$this->invoice->id);
return (new MailMessage)
->subject('Invoice Paid')
->markdown('mail.invoice.paid', ['url' => $url]);
}
メッセージの作成
Markdownメール通知は、Laravelの事前作成された通知コンポーネントを活用しながら、通知を簡単に構築できるようにするBladeコンポーネントとMarkdown構文の組み合わせを使用します:
⟨x-mail::message⟩
# 請求書が支払われました
請求書が支払われました!
⟨x-mail::button :url="$url"⟩
請求書を表示
⟨/x-mail::button⟩
ありがとうございます、⟨br⟩
{{ config('app.name') }}
⟨/x-mail::message⟩
ボタンコンポーネント
ボタンコンポーネントは、中央に配置されたボタンリンクをレンダリングします。このコンポーネントは、url
とオプションのcolor
の2つの引数を受け取ります。サポートされている色はprimary
、green
、およびred
です。通知にボタンコンポーネントを好きなだけ追加できます:
<x-mail::button :url="$url" color="green">
View Invoice
</x-mail::button>
パネルコンポーネント
パネルコンポーネントは、通知の残りの部分とは少し異なる背景色を持つパネル内に指定されたテキストブロックをレンダリングします。これにより、特定のテキストブロックに注意を引くことができます:
<x-mail::panel>
This is the panel content.
</x-mail::panel>
テーブルコンポーネント
テーブルコンポーネントは、MarkdownテーブルをHTMLテーブルに変換することができます。このコンポーネントは、Markdownテーブルをその内容として受け取ります。テーブル列の配置は、デフォルトのMarkdownテーブル配置構文を使用してサポートされています:
<x-mail::table>
| Laravel | Table | Example |
| ------------- | :-----------: | ------------: |
| Col 2 is | Centered | $10 |
| Col 3 is | Right-Aligned | $20 |
</x-mail::table>
コンポーネントのカスタマイズ
すべてのMarkdown通知コンポーネントをカスタマイズのためにアプリケーションにエクスポートできます。コンポーネントをエクスポートするには、vendor:publish
Artisanコマンドを使用してlaravel-mail
アセットタグを公開します:
php artisan vendor:publish --tag=laravel-mail
このコマンドは、Markdownメールコンポーネントをresources/views/vendor/mail
ディレクトリに公開します。mail
ディレクトリには、各利用可能なコンポーネントのそれぞれの表現を含むhtml
およびtext
ディレクトリが含まれます。これらのコンポーネントを自由にカスタマイズできます。
CSSのカスタマイズ
コンポーネントをエクスポートした後、resources/views/vendor/mail/html/themes
ディレクトリにはdefault.css
ファイルが含まれます。このファイル内のCSSをカスタマイズすると、スタイルがMarkdown通知のHTML表現内に自動的にインライン化されます。
LaravelのMarkdownコンポーネント用にまったく新しいテーマを構築したい場合は、html/themes
ディレクトリ内にCSSファイルを配置できます。CSSファイルの名前を付けて保存した後、theme
構成ファイルのmail
オプションを新しいテーマの名前に一致するように更新します。
個々の通知のテーマをカスタマイズするには、通知のメールメッセージを構築する際にtheme
メソッドを呼び出します。theme
メソッドは、通知を送信する際に使用されるテーマの名前を受け取ります:
/**
* Get the mail representation of the notification.
*/
public function toMail(object $notifiable): MailMessage
{
return (new MailMessage)
->theme('invoice')
->subject('Invoice Paid')
->markdown('mail.invoice.paid', ['url' => $url]);
}
データベース通知
前提条件
テーブルをクエリして、アプリケーションのユーザーインターフェースに通知を表示できます。しかし、その前に、通知を保持するためのデータベーステーブルを作成する必要があります。`````make:notifications-table`````コマンドを使用して、適切なテーブルスキーマを持つ[migration](/read/laravel-11-x/9d423b761ce26264.md)を生成できます:
``````shell
php artisan make:notifications-table
php artisan migrate
`
通知可能なモデルがUUIDまたはULIDプライマリキーを使用している場合、通知テーブルマイグレーションでmorphs
メソッドをuuidMorphs
またはulidMorphs
に置き換える必要があります。
データベース通知のフォーマット
通知がデータベーステーブルに保存されることをサポートしている場合、通知クラスにtoDatabase
またはtoArray
メソッドを定義する必要があります。このメソッドは、$notifiable
エンティティを受け取り、プレーンPHP配列を返す必要があります。返された配列はJSONとしてエンコードされ、data
列のnotifications
テーブルに保存されます。toArray
メソッドの例を見てみましょう:
/**
* Get the array representation of the notification.
*
* @return array<string, mixed>
*/
public function toArray(object $notifiable): array
{
return [
'invoice_id' => $this->invoice->id,
'amount' => $this->invoice->amount,
];
}
通知がアプリケーションのデータベースに保存されると、type
列は通知のクラス名で埋められます。ただし、この動作をカスタマイズするには、通知クラスにdatabaseType
メソッドを定義できます:
/**
* Get the notification's database type.
*
* @return string
*/
public function databaseType(object $notifiable): string
{
return 'invoice-paid';
}
toDatabase vs. toArray
<a name="accessing-the-notifications"></a>
### 通知へのアクセス
通知がデータベースに保存されると、通知可能なエンティティからそれらにアクセスする便利な方法が必要です。Laravelのデフォルトの`````App\Models\User`````モデルに含まれる`````Illuminate\Notifications\Notifiable`````トレイトには、エンティティの通知を返す`````notifications````` [Eloquentリレーションシップ](/read/laravel-11-x/3c1a7b9119505b21.md)が含まれています。通知を取得するには、このメソッドに他のEloquentリレーションシップと同様にアクセスできます。デフォルトでは、通知は`````created_at`````タイムスタンプでソートされ、最も最近の通知がコレクションの先頭に表示されます:
``````php
$user = App\Models\User::find(1);
foreach ($user->notifications as $notification) {
echo $notification->type;
}
`
「未読」通知のみを取得したい場合は、unreadNotifications
リレーションシップを使用できます。これらの通知もcreated_at
タイムスタンプでソートされ、最も最近の通知がコレクションの先頭に表示されます:
$user = App\Models\User::find(1);
foreach ($user->unreadNotifications as $notification) {
echo $notification->type;
}
JavaScriptクライアントから通知にアクセスするには、アプリケーションの通知コントローラを定義し、現在のユーザーなどの通知可能なエンティティの通知を返す必要があります。その後、JavaScriptクライアントからそのコントローラのURLにHTTPリクエストを行うことができます。
通知を既読としてマークする
通常、ユーザーが通知を表示したときに通知を「既読」とマークしたいと思うでしょう。Illuminate\Notifications\Notifiable
トレイトは、通知のデータベースレコードのread_at
列を更新するmarkAsRead
メソッドを提供します:
$user = App\Models\User::find(1);
foreach ($user->unreadNotifications as $notification) {
$notification->markAsRead();
}
ただし、各通知をループする代わりに、通知のコレクションに対してmarkAsRead
メソッドを直接使用できます:
$user->unreadNotifications->markAsRead();
データベースから取得せずに、すべての通知を既読としてマークするために一括更新クエリを使用することもできます:
$user = App\Models\User::find(1);
$user->unreadNotifications()->update(['read_at' => now()]);
通知をテーブルから完全に削除するためにdelete
することができます:
$user->notifications()->delete();
通知のブロードキャスト
前提条件
通知をブロードキャストする前に、Laravelのイベントブロードキャスティングサービスを構成し、理解しておく必要があります。イベントブロードキャスティングは、JavaScript駆動のフロントエンドからサーバー側のLaravelイベントに反応する方法を提供します。
ブロードキャスト通知のフォーマット
``````php
use Illuminate\Notifications\Messages\BroadcastMessage;
/**
* Get the broadcastable representation of the notification.
*/
public function toBroadcast(object $notifiable): BroadcastMessage
{
return new BroadcastMessage([
'invoice_id' => $this->invoice->id,
'amount' => $this->invoice->amount,
]);
}
`
ブロードキャストキューの構成
すべてのブロードキャスト通知は、ブロードキャストのためにキューに入れられます。ブロードキャスト操作のために使用されるキュー接続またはキュー名を構成したい場合は、onConnection
およびonQueue
メソッドをBroadcastMessage
で使用できます:
return (new BroadcastMessage($data))
->onConnection('sqs')
->onQueue('broadcasts');
通知タイプのカスタマイズ
指定するデータに加えて、すべてのブロードキャスト通知には、通知の完全なクラス名を含むtype
フィールドもあります。通知type
をカスタマイズしたい場合は、通知クラスにbroadcastType
メソッドを定義できます:
/**
* Get the type of the notification being broadcast.
*/
public function broadcastType(): string
{
return 'broadcast.message';
}
通知のリスニング
通知は、{notifiable}.{id}
形式を使用してプライベートチャネルでブロードキャストされます。したがって、App\Models\User
インスタンスに ID 1
を持つ通知を送信する場合、通知は App.Models.User.1
プライベートチャネルでブロードキャストされます。Laravel Echo を使用すると、notification
メソッドを使用してチャネルで通知を簡単にリスニングできます:
Echo.private('App.Models.User.' + userId)
.notification((notification) => {
console.log(notification.type);
});
通知チャネルのカスタマイズ
エンティティのブロードキャスト通知がブロードキャストされるチャネルをカスタマイズしたい場合は、通知可能なエンティティに receivesBroadcastNotificationsOn
メソッドを定義できます:
<?php
namespace App\Models;
use Illuminate\Broadcasting\PrivateChannel;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use Notifiable;
/**
* The channels the user receives notification broadcasts on.
*/
public function receivesBroadcastNotificationsOn(): string
{
return 'users.'.$this->id;
}
}
SMS通知
前提条件
LaravelでSMS通知を送信するには、Vonage(以前はNexmoとして知られていました)を使用します。Vonageを介して通知を送信する前に、laravel/vonage-notification-channel
および guzzlehttp/guzzle
パッケージをインストールする必要があります:
composer require laravel/vonage-notification-channel guzzlehttp/guzzle
このパッケージには 設定ファイル が含まれています。ただし、この設定ファイルを自分のアプリケーションにエクスポートする必要はありません。VONAGE_KEY
および VONAGE_SECRET
環境変数を使用して、Vonageの公開キーと秘密キーを定義できます。
キーを定義した後、SMSメッセージがデフォルトで送信される電話番号を定義する VONAGE_SMS_FROM
環境変数を設定する必要があります。この電話番号は、Vonageのコントロールパネル内で生成できます:
VONAGE_SMS_FROM=15556666666
SMS通知のフォーマット
通知がSMSとして送信されることをサポートしている場合は、通知クラスに toVonage
メソッドを定義する必要があります。このメソッドは $notifiable
エンティティを受け取り、Illuminate\Notifications\Messages\VonageMessage
インスタンスを返す必要があります:
use Illuminate\Notifications\Messages\VonageMessage;
/**
* Get the Vonage / SMS representation of the notification.
*/
public function toVonage(object $notifiable): VonageMessage
{
return (new VonageMessage)
->content('Your SMS message content');
}
Unicodeコンテンツ
SMSメッセージにUnicode文字が含まれる場合は、unicode
メソッドを呼び出して VonageMessage
インスタンスを構築する必要があります:
use Illuminate\Notifications\Messages\VonageMessage;
/**
* Get the Vonage / SMS representation of the notification.
*/
public function toVonage(object $notifiable): VonageMessage
{
return (new VonageMessage)
->content('Your unicode message')
->unicode();
}
「From」番号のカスタマイズ
通知を、VONAGE_SMS_FROM
環境変数で指定された電話番号とは異なる電話番号から送信したい場合は、from
メソッドを VonageMessage
インスタンスで呼び出すことができます:
use Illuminate\Notifications\Messages\VonageMessage;
/**
* Get the Vonage / SMS representation of the notification.
*/
public function toVonage(object $notifiable): VonageMessage
{
return (new VonageMessage)
->content('Your SMS message content')
->from('15554443333');
}
クライアントリファレンスの追加
ユーザー、チーム、またはクライアントごとのコストを追跡したい場合は、通知に「クライアントリファレンス」を追加できます。Vonageは、このクライアントリファレンスを使用してレポートを生成できるため、特定の顧客のSMS使用状況をよりよく理解できます。クライアントリファレンスは、最大40文字の任意の文字列にできます:
use Illuminate\Notifications\Messages\VonageMessage;
/**
* Get the Vonage / SMS representation of the notification.
*/
public function toVonage(object $notifiable): VonageMessage
{
return (new VonageMessage)
->clientReference((string) $notifiable->id)
->content('Your SMS message content');
}
SMS通知のルーティング
Vonageの通知を適切な電話番号にルーティングするには、通知可能なエンティティに routeNotificationForVonage
メソッドを定義します:
<?php
namespace App\Models;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Illuminate\Notifications\Notification;
class User extends Authenticatable
{
use Notifiable;
/**
* Route notifications for the Vonage channel.
*/
public function routeNotificationForVonage(Notification $notification): string
{
return $this->phone_number;
}
}
Slack通知
前提条件
Slack通知を送信する前に、Composerを介してSlack通知チャネルをインストールする必要があります:
composer require laravel/slack-notification-channel
さらに、Slackワークスペース用に Slackアプリ を作成する必要があります。
アプリが作成された同じSlackワークスペースに通知を送信するだけの場合は、アプリが chat:write
、chat:write.public
、および chat:write.customize
スコープを持っていることを確認する必要があります。これらのスコープは、Slack内の「OAuth & Permissions」アプリ管理タブから追加できます。
次に、アプリの「Bot User OAuth Token」をコピーし、アプリケーションの services.php
設定ファイル内の slack
設定配列に配置します。このトークンは、Slack内の「OAuth & Permissions」タブで見つけることができます:
'slack' => [
'notifications' => [
'bot_user_oauth_token' => env('SLACK_BOT_USER_OAUTH_TOKEN'),
'channel' => env('SLACK_BOT_USER_DEFAULT_CHANNEL'),
],
],
アプリの配布
アプリケーションが、アプリケーションのユーザーが所有する外部Slackワークスペースに通知を送信する場合、アプリをSlack経由で「配布」する必要があります。アプリの配布は、Slack内のアプリの「配布管理」タブから管理できます。アプリが配布されると、Socialite を使用して、アプリケーションのユーザーに代わって Slack Botトークンを取得できます。
Slack通知のフォーマット
通知がSlackメッセージとして送信されることをサポートしている場合は、通知クラスに toSlack
メソッドを定義する必要があります。このメソッドは $notifiable
エンティティを受け取り、Illuminate\Notifications\Slack\SlackMessage
インスタンスを返す必要があります。SlackのBlock Kit APIを使用してリッチ通知を構築できます。次の例は、SlackのBlock Kitビルダーでプレビューできます:
use Illuminate\Notifications\Slack\BlockKit\Blocks\ContextBlock;
use Illuminate\Notifications\Slack\BlockKit\Blocks\SectionBlock;
use Illuminate\Notifications\Slack\BlockKit\Composites\ConfirmObject;
use Illuminate\Notifications\Slack\SlackMessage;
/**
* Get the Slack representation of the notification.
*/
public function toSlack(object $notifiable): SlackMessage
{
return (new SlackMessage)
->text('One of your invoices has been paid!')
->headerBlock('Invoice Paid')
->contextBlock(function (ContextBlock $block) {
$block->text('Customer #1234');
})
->sectionBlock(function (SectionBlock $block) {
$block->text('An invoice has been paid.');
$block->field("*Invoice No:*\n1000")->markdown();
$block->field("*Invoice Recipient:*\n")->markdown();
})
->dividerBlock()
->sectionBlock(function (SectionBlock $block) {
$block->text('Congratulations!');
});
}
Slackのインタラクティビティ
SlackのBlock Kit通知システムは、ユーザーインタラクションを処理するための強力な機能を提供します。これらの機能を利用するには、Slackアプリで「インタラクティビティ」を有効にし、アプリケーションによって提供されるURLを指す「リクエストURL」を設定する必要があります。これらの設定は、Slack内の「インタラクティビティ & ショートカット」アプリ管理タブから管理できます。
次の例では、actionsBlock
メソッドを利用して、Slackは「リクエストURL」にPOST
リクエストを送信し、ボタンをクリックしたSlackユーザー、クリックされたボタンのIDなどを含むペイロードを送信します。アプリケーションは、そのペイロードに基づいて実行するアクションを決定できます。また、リクエストを検証して、Slackによって行われたことを確認する必要があります:
use Illuminate\Notifications\Slack\BlockKit\Blocks\ActionsBlock;
use Illuminate\Notifications\Slack\BlockKit\Blocks\ContextBlock;
use Illuminate\Notifications\Slack\BlockKit\Blocks\SectionBlock;
use Illuminate\Notifications\Slack\SlackMessage;
/**
* Get the Slack representation of the notification.
*/
public function toSlack(object $notifiable): SlackMessage
{
return (new SlackMessage)
->text('One of your invoices has been paid!')
->headerBlock('Invoice Paid')
->contextBlock(function (ContextBlock $block) {
$block->text('Customer #1234');
})
->sectionBlock(function (SectionBlock $block) {
$block->text('An invoice has been paid.');
})
->actionsBlock(function (ActionsBlock $block) {
// ID defaults to "button_acknowledge_invoice"...
$block->button('Acknowledge Invoice')->primary();
// Manually configure the ID...
$block->button('Deny')->danger()->id('deny_invoice');
});
}
確認モーダル
ユーザーがアクションを実行する前に確認を求める必要がある場合は、ボタンを定義する際に confirm
メソッドを呼び出すことができます。confirm
メソッドは、メッセージと ConfirmObject
インスタンスを受け取るクロージャを受け入れます:
use Illuminate\Notifications\Slack\BlockKit\Blocks\ActionsBlock;
use Illuminate\Notifications\Slack\BlockKit\Blocks\ContextBlock;
use Illuminate\Notifications\Slack\BlockKit\Blocks\SectionBlock;
use Illuminate\Notifications\Slack\BlockKit\Composites\ConfirmObject;
use Illuminate\Notifications\Slack\SlackMessage;
/**
* Get the Slack representation of the notification.
*/
public function toSlack(object $notifiable): SlackMessage
{
return (new SlackMessage)
->text('One of your invoices has been paid!')
->headerBlock('Invoice Paid')
->contextBlock(function (ContextBlock $block) {
$block->text('Customer #1234');
})
->sectionBlock(function (SectionBlock $block) {
$block->text('An invoice has been paid.');
})
->actionsBlock(function (ActionsBlock $block) {
$block->button('Acknowledge Invoice')
->primary()
->confirm(
'Acknowledge the payment and send a thank you email?',
function (ConfirmObject $dialog) {
$dialog->confirm('Yes');
$dialog->deny('No');
}
);
});
}
Slackブロックの検査
構築しているブロックを迅速に検査したい場合は、dd
メソッドを SlackMessage
インスタンスで呼び出すことができます。dd
メソッドは、ペイロードと通知のプレビューをブラウザに表示するSlackのBlock Kit BuilderへのURLを生成してダンプします。true
をdd
メソッドに渡して、生のペイロードをダンプできます:
return (new SlackMessage)
->text('One of your invoices has been paid!')
->headerBlock('Invoice Paid')
->dd();
Slack通知のルーティング
Slack通知を適切なSlackチームとチャネルに送信するには、通知可能なモデルに routeNotificationForSlack
メソッドを定義します。このメソッドは、次の3つの値のいずれかを返すことができます:
null
- 通知自体で構成されたチャネルへのルーティングを遅延させます。通知を構築する際にto
メソッドを使用して、通知内でチャネルを構成できます。- 通知を送信するSlackチャネルを指定する文字列、例:
#support-channel
. - OAuthトークンとチャネル名を指定できる
SlackRoute
インスタンス、例:SlackRoute::make($this->slack_channel, $this->slack_token)
。このメソッドは、外部ワークスペースに通知を送信するために使用する必要があります。
たとえば、#support-channel
を routeNotificationForSlack
メソッドから返すと、通知はアプリケーションの services.php
設定ファイルにあるBot User OAuthトークンに関連付けられたワークスペースの #support-channel
チャネルに送信されます:
<?php
namespace App\Models;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Illuminate\Notifications\Notification;
class User extends Authenticatable
{
use Notifiable;
/**
* Route notifications for the Slack channel.
*/
public function routeNotificationForSlack(Notification $notification): mixed
{
return '#support-channel';
}
}
外部Slackワークスペースへの通知
外部Slackワークスペースに通知を送信する前に、Slackアプリを配布する必要があります。
もちろん、アプリケーションのユーザーが所有するSlackワークスペースに通知を送信したい場合がよくあります。そのためには、まずユーザーのSlack OAuthトークンを取得する必要があります。幸いなことに、Laravel Socialiteには、アプリケーションのユーザーをSlackで簡単に認証し、ボットトークンを取得できるSlackドライバーが含まれています。
ボットトークンを取得し、アプリケーションのデータベースに保存したら、SlackRoute::make
メソッドを利用して通知をユーザーのワークスペースにルーティングできます。さらに、アプリケーションは、ユーザーが通知を送信するチャネルを指定する機会を提供する必要があります:
<?php
namespace App\Models;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Slack\SlackRoute;
class User extends Authenticatable
{
use Notifiable;
/**
* Route notifications for the Slack channel.
*/
public function routeNotificationForSlack(Notification $notification): mixed
{
return SlackRoute::make($this->slack_channel, $this->slack_token);
}
}
通知のローカライズ
Laravelでは、HTTPリクエストの現在のロケールとは異なるロケールで通知を送信でき、通知がキューに入れられた場合はこのロケールを記憶します。
これを実現するために、Illuminate\Notifications\Notification
クラスは、希望する言語を設定する locale
メソッドを提供します。アプリケーションは、通知が評価されるときにこのロケールに変更し、評価が完了すると前のロケールに戻ります:
$user->notify((new InvoicePaid($invoice))->locale('es'));
複数の通知可能なエントリのローカライズも Notification
ファサードを介して実現できます:
Notification::locale('es')->send(
$users, new InvoicePaid($invoice)
);
ユーザーの好みのロケール
アプリケーションが各ユーザーの好みのロケールを保存することがあります。通知可能なモデルに HasLocalePreference
インターフェースを実装することで、Laravelにこの保存されたロケールを使用して通知を送信するよう指示できます:
use Illuminate\Contracts\Translation\HasLocalePreference;
class User extends Model implements HasLocalePreference
{
/**
* Get the user's preferred locale.
*/
public function preferredLocale(): string
{
return $this->locale;
}
}
インターフェースを実装すると、Laravelは自動的に通知やメールをモデルに送信する際に好みのロケールを使用します。したがって、このインターフェースを使用する場合は、locale
メソッドを呼び出す必要はありません:
$user->notify(new InvoicePaid($invoice));
テスト
Notification
ファサードの fake
メソッドを使用して、通知が送信されないようにすることができます。通常、通知の送信は、実際にテストしているコードとは無関係です。おそらく、Laravelに特定の通知を送信するよう指示したことを単に主張するだけで十分です。
Notification
ファサードの fake
メソッドを呼び出した後、ユーザーに送信されるよう指示された通知を主張し、通知が受け取ったデータを検査することもできます:
<?php
use App\Notifications\OrderShipped;
use Illuminate\Support\Facades\Notification;
test('orders can be shipped', function () {
Notification::fake();
// Perform order shipping...
// Assert that no notifications were sent...
Notification::assertNothingSent();
// Assert a notification was sent to the given users...
Notification::assertSentTo(
[$user], OrderShipped::class
);
// Assert a notification was not sent...
Notification::assertNotSentTo(
[$user], AnotherNotification::class
);
// Assert that a given number of notifications were sent...
Notification::assertCount(3);
});
<?php
namespace Tests\Feature;
use App\Notifications\OrderShipped;
use Illuminate\Support\Facades\Notification;
use Tests\TestCase;
class ExampleTest extends TestCase
{
public function test_orders_can_be_shipped(): void
{
Notification::fake();
// Perform order shipping...
// Assert that no notifications were sent...
Notification::assertNothingSent();
// Assert a notification was sent to the given users...
Notification::assertSentTo(
[$user], OrderShipped::class
);
// Assert a notification was not sent...
Notification::assertNotSentTo(
[$user], AnotherNotification::class
);
// Assert that a given number of notifications were sent...
Notification::assertCount(3);
}
}
assertSentTo
または assertNotSentTo
メソッドにクロージャを渡して、特定の「真実テスト」を通過する通知が送信されたことを主張できます。指定された真実テストを通過する通知が少なくとも1つ送信された場合、主張は成功します:
Notification::assertSentTo(
$user,
function (OrderShipped $notification, array $channels) use ($order) {
return $notification->order->id === $order->id;
}
);
オンデマンド通知
テストしているコードがオンデマンド通知を送信する場合、assertSentOnDemand
メソッドを介してオンデマンド通知が送信されたことをテストできます:
Notification::assertSentOnDemand(OrderShipped::class);
assertSentOnDemand
メソッドの2番目の引数としてクロージャを渡すことで、オンデマンド通知が正しい「ルート」アドレスに送信されたかどうかを確認できます:
Notification::assertSentOnDemand(
OrderShipped::class,
function (OrderShipped $notification, array $channels, object $notifiable) use ($user) {
return $notifiable->routes['mail'] === $user->email;
}
);
通知イベント
通知送信イベント
通知が送信されると、Illuminate\Notifications\Events\NotificationSending
イベントが通知システムによって発行されます。これには「通知可能」エンティティと通知インスタンス自体が含まれます。このイベントに対して、アプリケーション内でevent listenersを作成できます:
use Illuminate\Notifications\Events\NotificationSending;
class CheckNotificationStatus
{
/**
* Handle the given event.
*/
public function handle(NotificationSending $event): void
{
// ...
}
}
NotificationSending
イベントのイベントリスナーが false
メソッドから handle
を返す場合、通知は送信されません:
/**
* Handle the given event.
*/
public function handle(NotificationSending $event): bool
{
return false;
}
イベントリスナー内で、notifiable
、notification
、および channel
プロパティにアクセスして、通知の受信者や通知自体についての詳細を知ることができます:
/**
* Handle the given event.
*/
public function handle(NotificationSending $event): void
{
// $event->channel
// $event->notifiable
// $event->notification
}
通知送信イベント
通知が送信されると、Illuminate\Notifications\Events\NotificationSent
イベントが通知システムによって発行されます。これには「通知可能」エンティティと通知インスタンス自体が含まれます。このイベントに対して、アプリケーション内でevent listenersを作成できます:
use Illuminate\Notifications\Events\NotificationSent;
class LogNotification
{
/**
* Handle the given event.
*/
public function handle(NotificationSent $event): void
{
// ...
}
}
イベントリスナー内で、notifiable
、notification
、channel
、および response
プロパティにアクセスして、通知の受信者や通知自体についての詳細を知ることができます:
/**
* Handle the given event.
*/
public function handle(NotificationSent $event): void
{
// $event->channel
// $event->notifiable
// $event->notification
// $event->response
}
カスタムチャネル
Laravelにはいくつかの通知チャネルが付属していますが、他のチャネルを介して通知を配信するために独自のドライバーを作成したい場合があります。Laravelはそれを簡単にします。始めるには、send
メソッドを含むクラスを定義します。このメソッドは、$notifiable
と $notification
の2つの引数を受け取る必要があります。
send
メソッド内で、通知に対してチャネルによって理解されるメッセージオブジェクトを取得するために通知のメソッドを呼び出し、その後、$notifiable
インスタンスに通知を送信する方法を自由に選択できます:
<?php
namespace App\Notifications;
use Illuminate\Notifications\Notification;
class VoiceChannel
{
/**
* Send the given notification.
*/
public function send(object $notifiable, Notification $notification): void
{
$message = $notification->toVoice($notifiable);
// Send notification to the $notifiable instance...
}
}
通知チャネルクラスが定義されたら、任意の通知の via
メソッドからクラス名を返すことができます。この例では、通知の toVoice
メソッドが音声メッセージを表すために選択したオブジェクトを返すことができます。たとえば、これらのメッセージを表すために独自の VoiceMessage
クラスを定義することができます:
<?php
namespace App\Notifications;
use App\Notifications\Messages\VoiceMessage;
use App\Notifications\VoiceChannel;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Notification;
class InvoicePaid extends Notification
{
use Queueable;
/**
* Get the notification channels.
*/
public function via(object $notifiable): string
{
return VoiceChannel::class;
}
/**
* Get the voice representation of the notification.
*/
public function toVoice(object $notifiable): VoiceMessage
{
// ...
}
}